In Laravel, you can use the pivot
method to access and manipulate the intermediate table data of a many-to-many relationship. To store data in the pivot table, you can follow these steps:
1. Define the many-to-many relationship between your models using the belongsToMany method.
class User extends Model
{
public function roles()
{
return $this->belongsToMany(Role::class)->withPivot('permission');
}
}
class Role extends Model
{
public function users()
{
return $this->belongsToMany(User::class)->withPivot('permission');
}
}
2. To store data in the pivot table, you can attach the related models using the attach
method, passing an array of additional data as the second argument.
$user = User::find(1);
$role = Role::find(2);
$user->roles()->attach($role, ['permission' => 'read']);
3. You can also update the data in the pivot table using the updateExistingPivot method.
$user = User::find(1);
$role = Role::find(2);
$user->roles()->updateExistingPivot($role->id, ['permission' => 'write']);
4. You can retrieve the data stored in the pivot table using the pivot attribute.
$user = User::find(1);
foreach ($user->roles as $role) {
echo $role->pivot->permission;
}
Note: In the example above, we added withPivot method in both belongsToMany relationships to allow us to access the permission field in the pivot table.
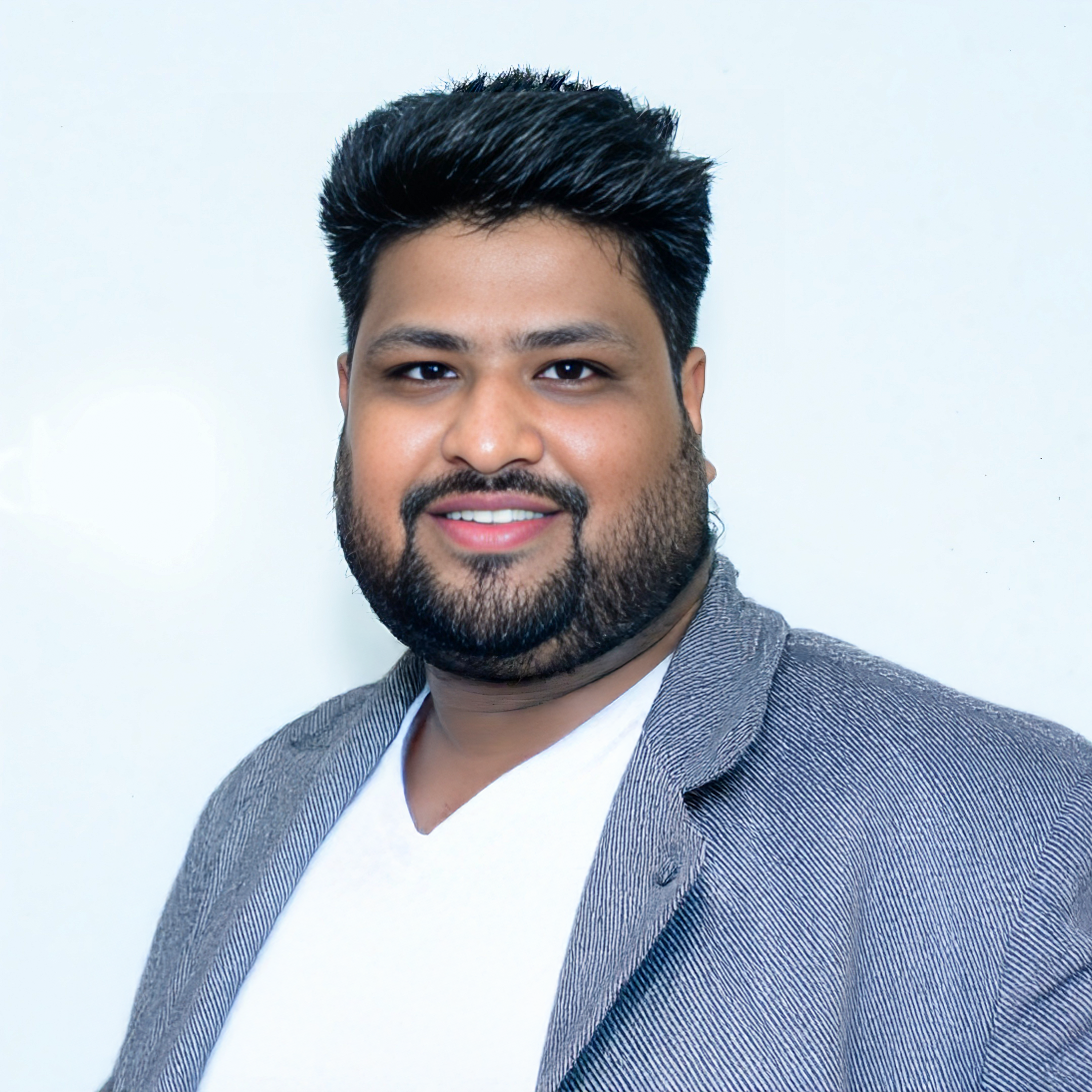
Hello All,
Aman Mishra has years of experience in the IT industry. His passion for helping people in all aspects of mobile app development. Therefore, He write several blogs that help the readers to get the appropriate information about mobile app development trends, technology, and many other aspects.In addition to providing mobile app development services in USA, he also provides maintenance & support services for businesses of all sizes. He tried to solve all their readers’ queries and ensure that the given information would be helpful for them.